Potentiometers are essential components in electronics, often used for adjusting signal levels, controlling devices, and measuring resistance. Their versatility makes them invaluable in applications ranging from simple dimmer switches to advanced input devices for microcontroller projects. In this guide, we explore ESP32 Potentiometer Reading using MicroPython, delving into the ADC capabilities of the ESP32, the mathematical formulas involved, and practical applications for controlling peripherals like LEDs.
Learning how to read analog signals with the ESP32 opens doors to a wide range of sensor-based projects. By understanding the principles behind ADC and implementing efficient code, you can transform raw data into meaningful outputs, enabling projects that respond dynamically to environmental changes. Whether you’re a beginner or an experienced developer, mastering these techniques is a stepping stone to more complex applications.
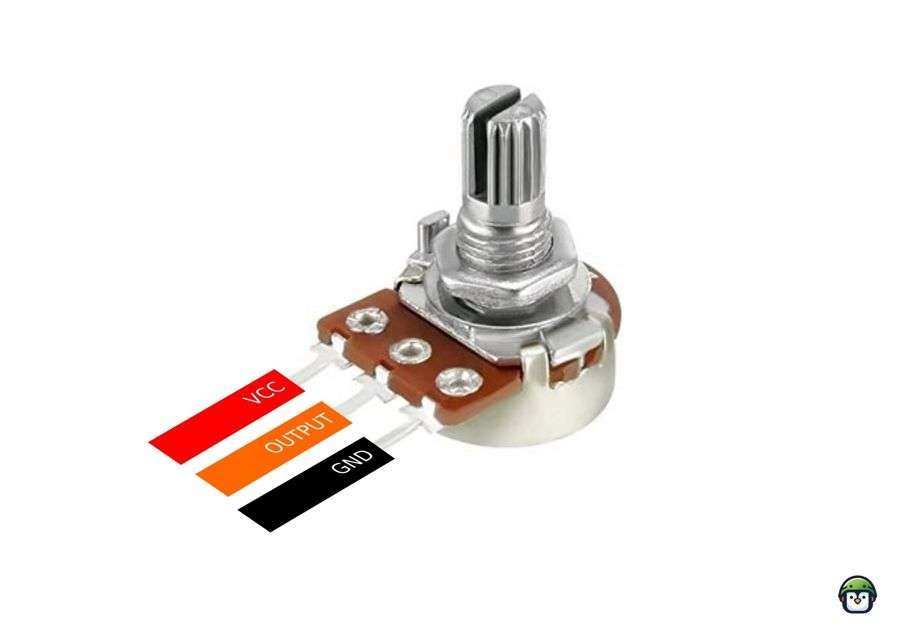
Table of Contents
Understanding ADC on the ESP32
The ESP32 features built-in ADC (Analog-to-Digital Converter) modules, which allow it to interpret analog signals by converting them into digital values. ADCs work by sampling the voltage level of an analog input and assigning it a numerical value, typically within a predefined range. The ESP32’s ADC range is 0–4095 for 12-bit resolution.
ADC Applications
ADC is crucial in scenarios where sensors output analog signals, such as:
- Potentiometers: Measure resistance changes.
- Light Sensors (e.g., LDRs): Detect light intensity.
- Temperature Sensors (e.g., thermistors): Read temperature variations.
- Gas Sensors: Detect gas concentrations.
- Flex Sensors: Measure bending or pressure.
- Force-Sensitive Resistors (FSRs): Detect applied pressure.
Components and Setup
- ESP32 board
- Potentiometer
- Connecting wires
- Breadboard
Wiring Diagram
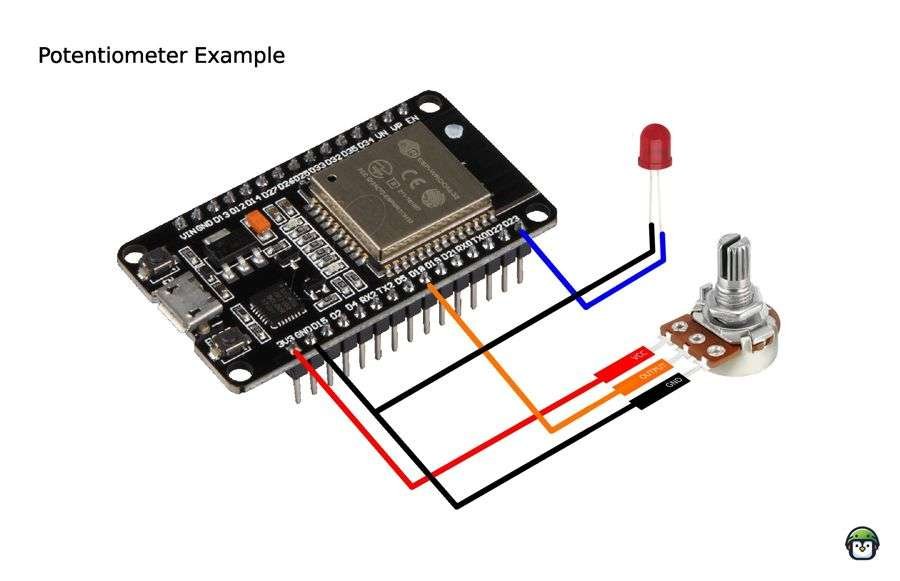
- Connect the potentiometer’s middle pin to ESP32’s ADC pin (e.g., GPIO18).
- Attach one outer pin to the 3.3V power supply.
- Attach the other outer pin to GND.
Here is a table based on the code provided, detailing the pinout for the potentiometer and LED setup:
Component | ESP32 Pin | Description |
---|---|---|
Potentiometer | GPIO18 | ADC pin to read the analog signal (middle pin) |
Potentiometer | 3.3V | Power supply (one outer pin) |
Potentiometer | GND | Ground (other outer pin) |
LED (PWM output) | GPIO23 | Controls LED brightness via PWM |
Reading Analog Values with MicroPython
Below is the code for ESP32 Potentiometer Reading, which also calculates the voltage and controls an LED’s brightness via PWM:
from time import sleep_ms
from machine import Pin, ADC, PWM
# Setup ADC and LED
pot = ADC(Pin(18))
pot.atten(ADC.ATTN_11DB) # Full voltage range (0-3.3V)
led = PWM(Pin(23), freq=5000)
while True:
pot_value = pot.read() # Read raw ADC value
voltage = pot_value * (3.3 / 4095) # Convert ADC to voltage
dc = int(0.24 * pot_value) # Calculate duty cycle
led.duty(dc) # Set LED brightness
print(f"Raw Value: {pot_value}, Voltage: {voltage:.2f}V, Duty Cycle: {dc}")
sleep_ms(50)
Decoding the Math Behind the Code
Voltage Conversion Formula
The formula for voltage conversion is:
- Raw ADC Value: Output from the ADC module.
- Reference Voltage: Typically 3.3V for the ESP32.
- Resolution: 12-bit ADC results in 4095 steps (2ⁱ² – 1).
For a raw ADC value of 2048, the voltage would be:
Duty Cycle Formula
The LED’s brightness is controlled using a PWM duty cycle calculated as:
The multiplier “0.24” ensures that the duty cycle remains within the allowable PWM range (0-1023 for the ESP32). Without scaling, raw ADC values (up to 4095) would exceed the PWM range, causing incorrect behavior.
Basically the duty cycle is is 24% of the POT value.
Practical Applications
This setup demonstrates how ESP32 Potentiometer Reading can be used to:
- Adjust LED brightness.
- Control motor speeds.
- Create user-friendly input devices like sliders.
Conclusion
ESP32 Potentiometer Reading is a fundamental skill for anyone working with analog sensors and ESP32. The ability to interpret analog signals using the ESP32’s ADC expands your project’s potential, allowing interaction with an extensive range of sensors. From potentiometers to light and temperature sensors, ADC unlocks opportunities to build responsive and innovative solutions.
By mastering these basics, you can take on advanced challenges in IoT, robotics, and automation. Dive deeper into sensor-based projects and experiment with other analog components to enhance your technical expertise and creativity.
Leave a Reply