Limit switches are essential components in various electronic and mechanical systems, used for detecting the position of a moving part. In ESP32-based projects, Limit Switches as Sensors can be employed to trigger events or limit motion, providing crucial feedback in automation systems. These switches are easy to integrate with the ESP32 microcontroller, which allows for the development of robust control systems in robotics, home automation, and more. In this guide, we will explore how to use Limit Switches as Sensors in ESP32 applications, covering their wiring, basic functionality, and practical use cases.
Limit switches typically consist of a mechanical actuator that responds to physical movement, opening or closing a circuit when a preset position is reached. In an ESP32 application, these switches can serve a variety of functions, such as detecting the end of a motor’s movement or acting as triggers for specific actions. Their simplicity, reliability, and versatility make them an excellent choice for numerous applications, including automation, robotics, and industrial control.
Table of Contents
How It Works
Limit switches typically contain a mechanical actuator that responds to external pressure or movement. When the actuator is pressed, it either closes or opens a circuit, depending on the design of the switch.
- Normally Closed (NC) Limit Switch: In contrast, a normally closed limit switch has a closed circuit by default, and pressing the actuator opens the circuit, signaling that contact has been lost or the limit has been exceeded.
- Normally Open (NO) Limit Switch: In its default state, the circuit remains open (off). When the switch is pressed, it closes the circuit, sending a signal to the system indicating that the limit has been reached or contact has been made.
Wiring Limit Switches as Sensors to ESP32
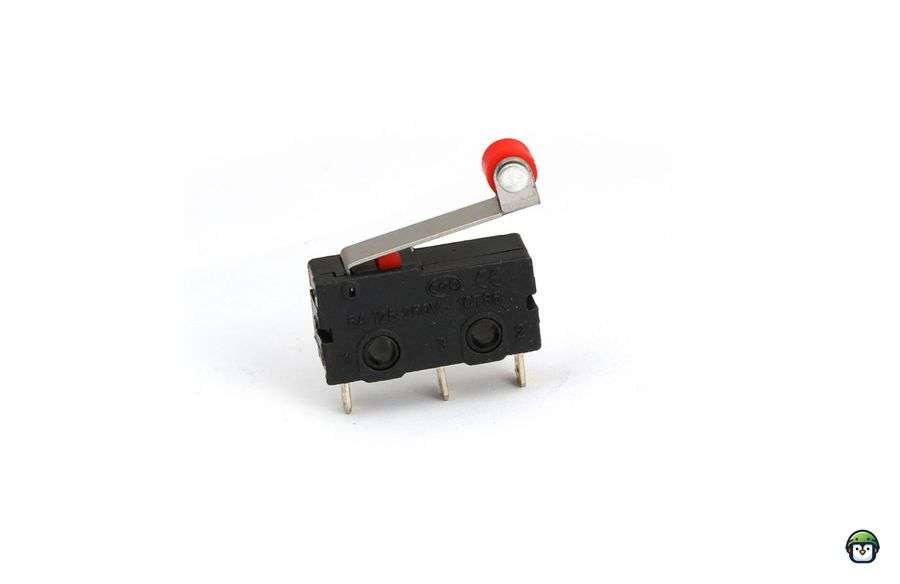
Before diving into applications, it’s essential to understand how to wire a Limit Switch as Sensor to the ESP32. The simplest setup involves connecting the limit switch to one of the ESP32’s GPIO (General Purpose Input/Output) pins.
- Limit Switch as Sensor Connections:
- One terminal of the limit switch connects to a digital GPIO pin on the ESP32 (e.g., GPIO 14).
- The other terminal of the limit switch connects to GND (Ground) or 3.3V, depending on the type of switch (normally open or normally closed).
- If you’re using a normally open (NO) Limit Switch as Sensor, the circuit will only be closed when the switch is activated (pressed or triggered by movement).
- Pull-up/Pull-down Resistor:
Most digital inputs on the ESP32 are configured with an internal pull-up or pull-down resistor, which can be enabled in software. For a normally open switch, you’ll want to enable the internal pull-up resistor to ensure the input pin is pulled to HIGH when the switch is not triggered.
Basic Limit Switches as Sensors Functionality with ESP32
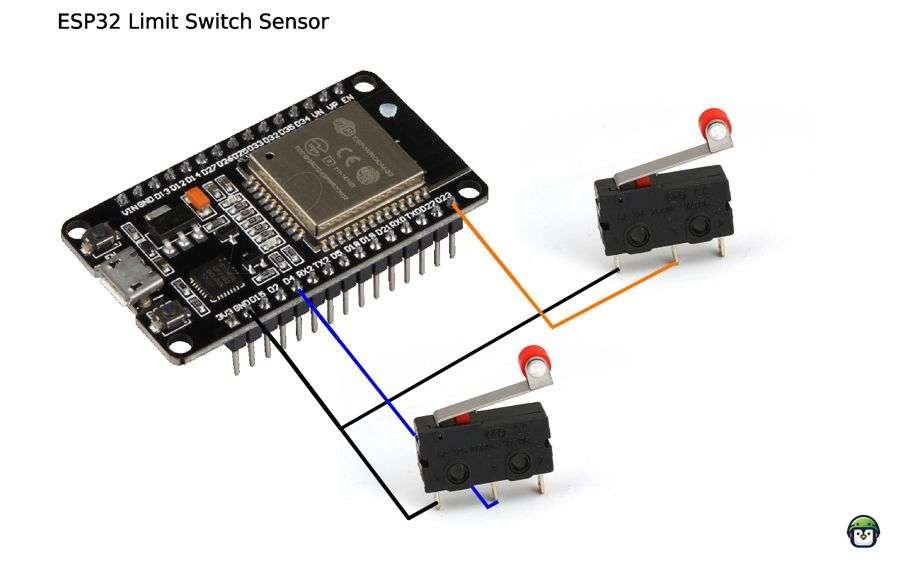
Limit Switches as Sensors are typically used in two ways: either as an input trigger to signal the microcontroller or as part of a feedback system for controlling motor or actuator movement. In the ESP32, you can monitor the state of a Limit Switch as Sensor through its GPIO pin. When the Limit Switch as Sensor is pressed or triggered, it will either send a LOW signal (in a normally open setup with a pull-up resistor) or a HIGH signal (in a normally closed setup with a pull-down resistor).
Here is a simple example of how to read the state of a Limit Switch as Sensor in MicroPython:
from machine import Pin
import time
# Define the GPIO pin for the limit switch
limit_switch1 = Pin(23, Pin.IN, Pin.PULL_DOWN) # GPIO23 with internal pull-up resistor
limit_switch2 = Pin(4, Pin.IN, Pin.PULL_DOWN) # GPIO4 with internal pull-up resistor
while True:
if limit_switch1.value() == 1: # The switch is triggered
print("Limit switch 1 activated")
else:
print("Limit switch 1not activated")
if limit_switch2.value() == 1: # The switch is triggered
print("Limit switch 2 activated")
else:
print("Limit switch 2 not activated")
time.sleep(0.1)
In this example, GPIO 14 is used to read the state of the Limit Switch as Sensor. The code checks if the switch is activated by detecting whether the value is LOW (indicating that the switch has been triggered). The program then prints a message to the console based on the switch’s state.
Practical Use Cases for Limit Switches as Sensors
Limit Switches as Sensors have various applications in ESP32 projects, providing feedback for automated systems. Below are a few examples where Limit Switches as Sensors can play a vital role:
- Motorized Doors:
Limit Switches as Sensors can detect when a motorized door reaches its fully open or closed position, preventing over-travel and protecting the motor from damage. The ESP32 can control the motor while using Limit Switches as Sensors to halt movement when the door reaches the desired position. - Robotic Arms:
In robotic arm applications, Limit Switches as Sensors are used to detect the extreme positions of the arm’s joints. When the arm reaches the limit, the switch activates, allowing the ESP32 to stop the movement or change its direction. - Elevator Systems:
Limit Switches as Sensors are commonly used in elevator systems to detect the top and bottom floor positions. The ESP32 can use these sensors to control the elevator’s stopping mechanism, ensuring it doesn’t exceed the desired position. - 3D Printers:
Limit Switches as Sensors are often used in 3D printers to detect the position of the print head or bed. The ESP32 can use the Limit Switch as Sensor to calibrate the printer’s movements, ensuring accurate prints every time.
Benefits of Using Limit Switches as Sensors with ESP32
Using Limit Switches as Sensors in ESP32 applications brings several advantages:
- Reliability: Limit Switches as Sensors are mechanical components that provide a simple yet highly reliable way to detect position. They are less prone to failure compared to optical or magnetic sensors in certain environments.
- Cost-Effective: Limit Switches as Sensors are inexpensive and readily available, making them a budget-friendly choice for many automation and control projects.
- Ease of Use: Integrating Limit Switches as Sensors with the ESP32 is straightforward, requiring only basic wiring and simple programming to implement.
- Versatility: Limit Switches as Sensors can be used in various applications, from robotics to home automation systems, providing position feedback for more accurate control.
Conclusion
Incorporating Limit Switches as Sensors into your ESP32 projects is a practical and cost-effective solution for detecting positions and triggering actions. By understanding the basics of wiring and programming Limit Switches as Sensors, you can enhance your ESP32-based applications with reliable and accurate feedback mechanisms. Whether you’re building automation systems, robotics, or control systems, Limit Switches as Sensors provide a solid foundation for ensuring precision and safety in your projects.
By experimenting with Limit Switches as Sensors, you’ll be able to explore new possibilities in motion control and automation. Make sure to consider the types of switches (normally open vs. normally closed) and configure the ESP32 pins accordingly to suit your needs.
Leave a Reply