
In today’s world, speech-to-text technology has become essential in various applications, from transcribing meetings to enabling voice-activated devices. One of the powerful tools in this domain is the Whisper API, an open-source speech recognition system. In this article, we’ll guide you through the process of converting WAV files to text using the Whisper API, including installation steps, dependencies, and some common use cases.
Table of Contents
- Introduction to Whisper API
- Prerequisites
- Installation and Dependencies
- Converting WAV to Text Using Whisper API
- How It Works
- Use Cases
- Frequently Asked Questions (FAQs)
Introduction to Whisper API
The Whisper API is an advanced speech recognition model developed by OpenAI and made available as a free and open-source tool. It’s designed to understand and transcribe spoken language with high accuracy, making it a valuable tool for developers and researchers working on voice-activated applications, transcription services, and more.
Prerequisites
Before we start, ensure you have the following:
- Python installed on your system
- Basic knowledge of Python programming
- Internet connection to download dependencies
Installation and Dependencies
First, let’s install the necessary dependencies. We’ll need whisper
and pydub
for handling audio files. Install these using pip:
$ pip install openai-whisper pydub
Additionally, you might need ffmpeg
to handle audio conversions. You can install it using:
- Windows: Download the executable from FFmpeg website and add it to your PATH.
- macOS: Use Homebrew with the command
brew install ffmpeg
. - Linux: Use your package manager, e.g.,
sudo apt install ffmpeg
for Debian-based systems.
Converting WAV to Text Using Whisper API
Here’s a simple Python script to convert a WAV file to text using the Whisper API:
import whisper
from pydub import AudioSegment
def convert_wav_to_text(wav_file_path, output_text_file):
# Load and prepare the audio file
audio = AudioSegment.from_wav(wav_file_path)
audio = audio.set_channels(1)
audio.export("temp.wav", format="wav")
# Load Whisper model and transcribe
model = whisper.load_model("base")
result = model.transcribe("temp.wav")
# Save transcription to file
with open(output_text_file, 'w') as f:
f.write(result['text'])
print(f"Transcribed text written to {output_text_file}")
# Usage example
convert_wav_to_text("your_audio_file.wav", "transcribed_text.txt")
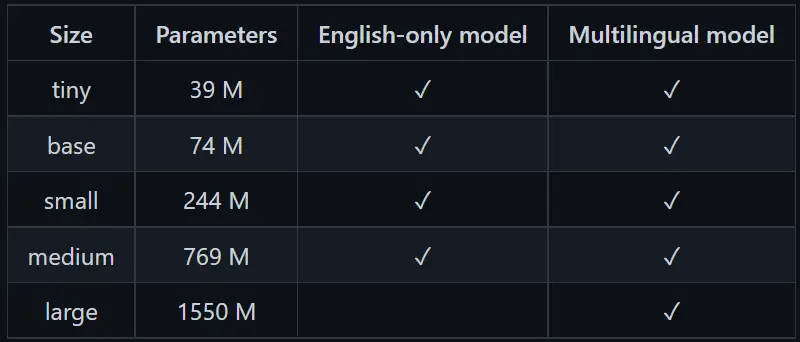
How It Works
- Audio Loading: We use
pydub
to load the WAV file and ensure it is in mono channel format, as Whisper API works best with single-channel audio. - Whisper Model Loading: We load the Whisper model using the
whisper
library. - Transcription: The model transcribes the audio file and prints the transcribed text.
Use Cases
- Meeting Transcription – Record your meetings and use this script to transcribe them, making it easy to reference and share minutes.
- Podcast Transcription – Convert podcast episodes into text for creating show notes or blog posts.
- Voice Command Applications – Implement voice commands in your applications by transcribing spoken words to text and processing them accordingly.
- Accessibility – Provide transcripts for audio content, making it accessible to individuals with hearing impairments.
Frequently Asked Questions (FAQs)
Q1: What audio formats does Whisper support?
Whisper primarily supports WAV files, but you can convert other audio formats to WAV using tools like ffmpeg
or pydub
.
Q2: How accurate is the Whisper API?
The accuracy of Whisper API depends on the quality of the audio and the clarity of the speech. It’s known for high accuracy in clear recordings.
Q3: Can Whisper handle multiple languages?
Yes, Whisper is designed to recognize and transcribe multiple languages.
Q4: Is Whisper API free to use?
Yes, the Whisper API is open-source and free to use.
Q5: What are the system requirements for running Whisper?
You need Python and the necessary libraries installed. Whisper works on all major operating systems, including Windows, macOS, and Linux.
With this guide, you should be able to convert WAV files to text using the Whisper API with ease. Whether you’re looking to transcribe meetings, podcasts, or implement voice commands, Whisper offers a powerful and flexible solution. Happy coding!
The Ultimate Guide to Termux: Mastering Automation, Customization, and Development on Android
Whether you’re looking to automate tasks, customize your environment, or develop cutting-edge applications, this guide has you covered. Start mastering Termux now and transform your Android device into a powerhouse of productivity and innovation. Don’t wait—grab your copy and start your journey to becoming a Termux pro!

Leave a Reply